Built-in Functions
You can embed raw javascript in your patterns
You can embed raw javascript in your patterns, by surrounding it with 'bee-stings' (i.e. wrap it in <% and %> )
A lot of people overlook this feature, even when they read about it, so I'll repeat it one more time, and put it in a blockquote
and and add emphasis
:
“You can embed raw javascript in your patterns”
This is very powerful. Your patterns can do everything javascript can do.
You rarely need all that power. Most of the time, all you need is a single function call, to a well-chosen function that manipulates text.
In the general help there are general examples, and below are examples specific to every "built-in" function. This is part of the "batteries included" aspect of Nimble Text. The functions you want are already loaded into the Functions
menu♦, ready to be added to your pattern.
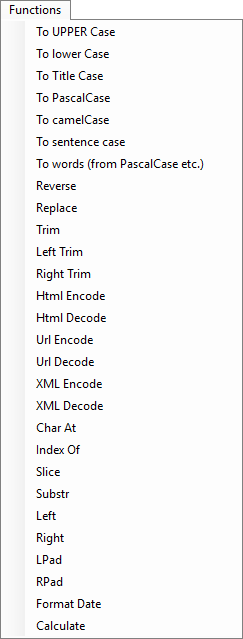
The 'Functions' menu.
♦ Functions menu is only present in the desktop version
The functions are now in groups, so it no longer looks like the long tall menu at right →
Any function that you "favorite" (⭐) will also be listed directly in the "top level" of the functions menu.
-
Case Functions
- toUpperCase
UPPERCASE
- toLowerCase
lowercase
- toTitleCase
Title Case
¤ - toPascalCase
PascalCase
¤ - toCamelCase
camelCase
¤ - toSentenceCase
Sentence case
¤ - toSnakeCase
snake_case
¤ - toKebabCase
kebab-case
¤ - toTrainCase
Train-Case
¤ - toVowelCase
vOwElcAsE
- toRandomCase
RaNdOMCase
- toWords (from PascalCase etc)
¤ 💡TIP You may need to use
toWords()
before using these functions. - toUpperCase
-
Date Functions (Powerful ones)
- dateFormat ♦
- toDate ♦
- reFormateDate ♦
- Encoding / Decoding
- Padding (left/right)
- Trimming (left/right)
- Substring (slice, left, right)
- All kinds of functions!
♦ These features are only available in the Desktop version. (Compare versions).
Case Functions
- toUpperCase
UPPERCASE
- toLowerCase
lowercase
- toTitleCase
Title Case
¤ - toPascalCase
PascalCase
¤ - toCamelCase
camelCase
¤ - toSentenceCase
Sentence case
¤ - toSnakeCase
snake_case
¤ - toKebabCase
kebab-case
¤ - toTrainCase
Train-Case
¤ - toVowelCase
vOwElcAsE
- toRandomCase
RaNdOMCase
- toWords (from PascalCase etc)
¤ 💡TIP You may need
to use toWords()
before using these functions.
toUpperCase
The built-in function toUpperCase
changes your text to UPPER CASE.
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
try
it →
<% $row.toUpperCase() %>
...would be:
ALL WORK AND NO PLAY MAKES JACK A DULL BOY
Commonly seen in internet forums, this casing style is recommended for when you are angry, and wishing to either really get your point across, or to ensure that you alienate the recipient of an email.
toLowerCase
The built-in function toLowerCase
changes your text to lower case.
If the input data said:
ALL WORK AND NO PLAY MAKES JACK A DULL BOY
...then the output of this pattern:
try
it →
<% $row.toLowerCase() %>
...would be:
all work and no play makes jack a dull boy
toTitleCase
The built-in function toTitleCase
changes your text to Title Case,
meaning that each word is capitalized.
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
try
it →
<% $row.toTitleCase() %>
...would be:
All Work And No Play Makes Jack A Dull Boy
toPascalCase
The built-in function toPascalCase
changes your text to PascalCase,
meaning that each word is capitalized and then spaces are removed.
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
try
it →
<% $row.toPascalCase() %>
...would be:
AllWorkAndNoPlayMakesJackADullBoy
toCamelCase
The built-in function toCamelCase
changes your text to camelCase
,
which is just like PascalCase
, but more camel-like. Technically, the difference
is that in camelCase
the first letter of the first word is lower-case. Thus PascalCase
is sometimes
called "Upper Camel Case" and this camelCase
could be called "lower Camel Case". It
is a small difference, but somehow worth an extra function.
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
try
it →
<% $row.toCamelCase() %>
...would be:
allWorkAndNoPlayMakesJackADullBoy
toSentenceCase
The built-in function toSentenceCase
changes your text to Sentence
case, meaning that the first word is capitalized, and all of the other words are
left alone.
If the input data was all lower case, and for example said:
all work and no play makes jack a dull boy
...then the output of this pattern:
try
it →
<% $row.toSentenceCase() %>
...would be:
All work and no play makes jack a dull boy
toRandomCase
The built-in function toRandomCase
randomly changes each letter to upper or lower case.
If the input data said:
All work and no play makes jack a dull boy All work and no play makes jack a dull boy All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.toRandomCase() %>
would be something like:
all WorK aND No PLAy MAkES jaCk a dULL BOY All WOrK AnD No plaY mAKeS Jack A Dull bOY alL WOrK anD no PlAY makeS JAck a DulL BOY
toSnakeCase
The built-in function toSnakeCase
converts words to "snake_case" - i.e. lower case with underscores between words.
If the input data said:
All work and no play makes jack a dull boy All work and no play makes jack a dull boy All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.toSnakeCase() %>
would be something like:
all_work_and_no_play_makes_jack_a_dull_boy all_work_and_no_play_makes_jack_a_dull_boy all_work_and_no_play_makes_jack_a_dull_boy
toKebabCase
The built-in function toKebabCase
converts words to "kebab-case" - i.e. lower case with hyphens between words.
If the input data said:
All work and no play makes jack a dull boy All work and no play makes jack a dull boy All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.toKebabCase() %>
would be something like:
all-work-and-no-play-makes-jack-a-dull-boy all-work-and-no-play-makes-jack-a-dull-boy all-work-and-no-play-makes-jack-a-dull-boy
toTrainCase
The built-in function toTrainCase
converts words to "Train-Case" - i.e. first letter of each word is captialized and the words are separatd by hyphens.
If the input data said:
All work and no play makes jack a dull boy All work and no play makes jack a dull boy All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.toTrainCase() %>
would be something like:
All-Work-And-No-Play-Makes-Jack-A-Dull-Boy All-Work-And-No-Play-Makes-Jack-A-Dull-Boy All-Work-And-No-Play-Makes-Jack-A-Dull-Boy
toVowelCase
The built-in function toVowelCase
converts words to "vOwElcAsE" - i.e. vowels are capitalized.
If the input data said:
All work and no play makes jack a dull boy All work and no play makes jack a dull boy All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.toVowelCase() %>
would be something like:
All wOrk And nO plAy mAkEs jAck A dUll bOy All wOrk And nO plAy mAkEs jAck A dUll bOy All wOrk And nO plAy mAkEs jAck A dUll bOy
toWords
The built-in function toWords
splits any PascalCase or camelCase words
into their constituent parts (by inserting spaces before each capital letter)
If the input data said:
PersonName ManagerRole
...then the output of this pattern:
try
it →
<% $row.toWords() %>
...would be:
Person Name Manager Role
reverse
The built-in function reverse
reverses the order of your text, for
that 'gone crazy' look.
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
try
it →
<% $row.reverse() %>
...would be:
yob llud a kcaj sekam yalp on dna krow llA
replace
The built-in function replace
lets you replace one value with another.
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
try
it →
<% $row.replace('a dull boy','an axe-wielding maniac') %>
...would be:
All work and no play makes jack an axe-wielding maniac
You can also perform multiple replaces, one after the other.
For example, this pattern:
try it →<% $row = $row.replace('a dull boy','an axe-wielding maniac'); $row = $row.replace('an axe','a teddy bear'); $row %>
Produces the result:
All work and no play makes jack a teddy bear-wielding maniac
Or, you can chain the replacements together, one after the other like so:
try it →<% $row .replace('a dull boy','an axe-wielding maniac') .replace('an axe','a teddy bear') %>
...for the same result as the previous example.
The 'replace' function is part of javascript, it isn't something extra that NimbleText has added on top (unlike the toPascalCase function, for example)
Replace can also take a regular expression as the first parameter.
For example you could turn all vowels into your favourite vowel sound ('oo'), with a pattern like this:
try
it →
<% $row.replace(/[aeiou]/ig,'oo') %>
Which produces the lyrically pleasing:
ooll woork oond noo plooy mookoos joock oo dooll booy
For a complete reference on using javascript's replace method, I recommend the Javascript Replace documentation at the Mozilla Developer Network.
htmlEncode
The built-in function htmlEncode
performs 'html Encoding' on your text.
If the input data said:
Ten < Twenty & is > Five
...then the output of this pattern:
<% $row.htmlEncode() %>
...would be:
Ten < Twenty & is > Five
htmlDecode
The built-in function htmlDecode
decodes text that has previously been
html encoded.
If the input data said:
Ten < Twenty & is > Five
...then the output of this pattern:
<% $row.htmlDecode() %>
...would be:
Ten < Twenty & is > Five
urlEncode
The built-in function urlEncode
lets you url Encode your text.
If the input data said:
http://google.com/
...then the output of this pattern:
<% $row.urlEncode() %>
...would be:
http%3A%2F%2Fgoogle.com%2F
Or, if the input data said:
A B C D E F
...then the output would be:
A%20B%20C%20D%20E%20F
urlDecode
The built-in function urlDecode
decodes text that has previously been
url encoded.
If the input data said:
http%3A%2F%2Fgoogle.com%2F
...then the output of this pattern:
<% $row.urlDecode() %>
...would be:
http://google.com/
Or if the input data said:
A%20B%20C%20D%20E%20F
...then the output would be:
A B C D E F
xmlEncode
The built-in function xmlEncode
encodes special characters that are
not permitted inside XML tags or attributes. Specifically it makes the following
changes:
From | To |
---|---|
< | < |
> | > |
" | " |
& | & |
' | ' |
If the input data said:
All <em>work</em> & 'no' play makes jack a "dull" boy
...then the output of this pattern:
<% $row.xmlEncode() %>
...would be:
All <em>work</em> & 'no' play makes jack a "dull" boy
xmlDecode
The built-in function xmlDecode
decodes special characters that were
encoded by an Xml Encoding process. Specifically it makes the following
changes:
From | To |
---|---|
< | < |
> | > |
" | " |
& | & |
' | ' |
If the input data said:
All <em>work</em> & 'no' play makes jack a "dull" boy
...then the output of this pattern:
<% $row.xmlDecode() %>
...would be:
All <em>work</em> & 'no' play makes jack a "dull" boy
split
The built-in function split
lets you separate a string into an array of substrings, at every instance of a separator. (The separator can be a single character, a string, or even a regular expression!)
If the input data said:
Mr Jones,2012-01 Professor Smith,2014-02
...then the output of this pattern:
<% $0.split(' ')[1] %>, <% $1.split('-')[0] %>
...would be:
Jones,2012 Smith,2014
See also: Mozilla's documentation about split.
charAt
The built-in function charAt
let you grab any character, by its index
number (starting at 0).
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.charAt(0) %>
...would be:
A
...because character 0 (the first character) is 'A'.
indexOf
The built-in function indexOf
returns the index (the location) of the first occurrence of the
specified string It is case sensitive.
Some programming languages call their indexOf
function 'find
', 'Pos
', 'Locate
' or 'InStr
' (see wikipedia string functions "find").
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.indexOf('no play') %>
...would be:
13
...because 'no play' appears starting at index 13.
lastIndexOf
The built-in function lastIndexOf
returns the index (the location) of the LAST occurrence of the
specified string. It is case sensitive.
Some programming languages call their lastIndexOf
function 'rfind
', 'rindex
', 'strrpos
' or 'InStrRev
' (see wikipedia string functions "rfind").
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.lastIndexOf('ll') %>
...would be:
36
...because the 'll' in "dull" is at index 36.
slice
The built-in function slice
returns a section of a string, from a starting
position up to an optional end position.
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.slice(4,8) %>
...would be:
work
Alternatively, you can specify a negative starting position to read from the end of the string.
Here is a table of examples:
Example | Result | Explanation |
---|---|---|
<% $row.slice(27) %> | jack a dull boy | everything after the first 27 characters |
<% $row.slice(-15) %> | jack a dull boy | everything after the (15th last) character |
<% $row.slice(27,31) %> | jack | everything from the 27th to 31st character |
<% $row.slice(27,-11) %> | jack | everything from the 27th to the (11th last) character |
<% $row.slice(-15,-11) %> | jack | everything from the (15th last) to the (11th last) character |
substr
The built-in function substr
returns a section of a string, from a starting
position, for a specified number of characters. It is similar to slice
, but instead of specifying the end index, you specify the number of characters to take.
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.substr(4,4) %>
...would be:
work
left
The built-in function left
allows you to take the first n characters from a string. It is provided for convenience to people with a background in SQL, or Visual Basic, or any platform that has a 'left' function. It is a simple wrapper on the substr function.
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.left(8) %>
...would be:
All work
right
The built-in function right
allows you to take the last n characters from a string. It is provided for convenience to people with a background in SQL, or Visual Basic, or any platform that has a 'right' function. It is a simple wrapper on the substr function.
If the input data said:
All work and no play makes jack a dull boy
...then the output of this pattern:
<% $row.right(10) %>
...would be:
a dull boy
lpad
The built-in function lpad
allows you to pad a string with characters on the left, to make a fixed-width value that is right-aligned. It is provided for convenience to people with a background in Oracle, Visual Basic, or any platform that has a 'left pad' function. It is commonly used with numeric values. Here's a detailed example.
If the input data said:
12,23 14,25
...then the output of this pattern:
x<% $0.lpad(7) %>x,y<% $1.lpad(7,'0') %>y
...would be:
x 12x,y0000023y x 14x,y0000025y
rpad
The built-in function rpad
allows you to pad a string with characters on the right, to make a fixed-width value that is left-aligned. It is provided for convenience to people with a background in Oracle, Visual Basic, or any platform that has a 'right pad' function. It is commonly used with non-numeric values. Here's a detailed example.
If the input data said:
1,3 2,25 3,128 4,545 5,613 6,723 7,840 8,1052
...then the output of this pattern:
<% ("Chapter " + $0).rpad(20,'.') %>pg <% $1.lpad(4,' ') %>
...would be:
Chapter 1...........pg 3 Chapter 2...........pg 25 Chapter 3...........pg 128 Chapter 4...........pg 545 Chapter 5...........pg 613 Chapter 6...........pg 723 Chapter 7...........pg 840 Chapter 8...........pg 1052
repeat
The built-in function Repeat
repeats a string as many times as you command.
If the input data said:
Hello
...then the output of this pattern:
<% $0.repeat(3) %>
...would be:
Hello Hello Hello
newGuid
♦ This feature is only available in the Desktop version. (Compare versions).
The built-in function newGuid will generate a new, random, guid
for you.
If the input data said:
1 2 3
...then the output of this pattern:
<% newGuid() %>
...would be something like:
32773762-427d-9c1f-fbe1-42cc66548fe1 f5842ffd-249c-68e5-4d45-21d75b4ebcca 66697ad2-8976-c491-f442-a89287fb14c3
It's unlikely you'd receive these exact guids. And every time you run the pattern you'll get different random guids.
To wrap the guid in {
and }
use this pattern:
{<% newGuid() %>}
...or this variation:
<% "{" + newGuid() + "}" %>
dateFormat
♦ This feature is only available in the Desktop version. (Compare versions).
The built-in function dateFormat (also Date.prototype.format
) allows you to format a date type, in whatever way you prefer. There is a separate help page that documents all of the ways you can format a date.
If the input data said:
2016-11-25
...then the output of this pattern:
<% $0.dateFormat('ddd, dd MMM yyyy') %>
...would be:
Fri, 25th Nov 2016.
toDate
♦ This feature is only available in the Desktop version. (Compare versions).
The built-in function toDate lets you parse a string and convert it into a date. To do this you need to specify a format. There is a separate help document to describe all the great things you can do with the toDate function.
If the input data said:
23/12/2021
...then the output of this pattern:
<% $0.toDate("d/M/yyyy") %>
...would depend on your timezone, but for me, I get this result:
Thu Dec 23 2021 00:00:00 GMT+1000 (E. Australia Standard Time)
And this function is best combined with the dateFormat function, which converts a date back into a string.
For example, if the input data said:
23/12/2021
...then the output of this pattern:
<% $0.toDate("d/M/yyyy").dateFormat("yyyy-MM-dd") %>
...would be:
2021-12-23
Thus, you can start with dates expressed in any format, and end up with dates in any other format.
Further help
You can also get general help, help on all the symbols and keywords, or on the built-in functions, filtering with a where clause, help with the powerful command-line automation, or applying custom formats to your dates and times.
- General help
- Symbols and Keywords
- Built-in Functions
- The 'Where' clause
- Date Time formatting
- Date Time parsing
- Command-Line Automation
- SQL Master Class
- HTML Master Class
- Popular Text Manipulations Made Easy «« bookmark this one
You need to purchase a license to unlock all the features in NimbleText.
If you haven't downloaded NimbleText yet, then for added power, privacy and versatility I sincerely think you should download it now.
Download NimbleText